Google is the most popular search engine and ranking high on Google’s search engine results pages (SERPs) is key for any website’s success. Tracking your website’s keyword rankings can be a time-consuming task, but with the help of Python, it can be automated. Python is a powerful programming language that can be used to automate tasks. In this article, I will show you how to track Google rankings using Python.
The code sample provided above is a simple Python program that can be used to track keyword rankings on Google. The program starts by importing the necessary modules, including CSV, time, selenium, and bs4. The selenium module is used to open up a web browser and access Google’s SERPs, while bs4 is used to parse the HTML from the page. The program then opens up a browser in incognito mode and imports the csv file containing the keywords to be tracked.
Next, the program loops through each keyword in the CSV file and visits Google’s search page for that keyword. It then waits for 10 seconds to allow the page to load before parsing the HTML with bs4. The program then excludes results from the classes “M8OgIe” and “ULSxyf” which are not relevant to our search.
Finally, the program finds the ranking position of the website by finding all elements with the “yuRUbf” class and looping through them until it finds a link that starts with the website URL. It then prints the keyword and its ranking position and exports the results to a CSV file.
Overall, tracking keyword rankings with Python is a simple and effective way to automate the process. The code sample provided above can be used as a starting point and can be easily modified to fit your needs.
Python code to check keyword rankings on Google
#importing the necessary modules
import csv
import time
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
from bs4 import BeautifulSoup
#opening the website in incognito mode
chrome_options = Options()
chrome_options.add_argument("--incognito")
driver = webdriver.Chrome(options=chrome_options)
#importing the keywords.csv file
csv_file = csv.reader(open('keywords.csv'))
for row in csv_file:
keyword = row[0]
driver.get('https://www.google.com/')
driver.get('https://www.google.com/search?q='+keyword+'&num=50')
time.sleep(5)
#exclude results from class 'M8OgIe' and 'ULSxyf' on google.com results
soup = BeautifulSoup(driver.page_source, 'lxml')
for item in soup.find_all('div', {'class': ['M8OgIe', 'ULSxyf']}):
item.decompose()
# Getting keyword ranking position results only from class 'yuRUbf' on Google.com
results = soup.find_all('div', {'class': 'yuRUbf'})
#finding the ranking position of the website https://www.example.com
ranking_position = 0
for result in results:
link = result.find('a').get('href')
if link.startswith('https://www.example.com'):
ranking_position = results.index(result) + 1
break
#printing the results
print('Keyword:', keyword, ' Ranking Position:', ranking_position)
#exporting the csv including all keywords with the date and time
with open('keyword_ranking_results.csv', 'a') as csv_file:
writer = csv.writer(csv_file)
writer.writerow([keyword, ranking_position, time.strftime('%Y-%m-%d %H:%M:%S')])
driver.quit()
Source: https://github.com/ramesh-s-bisht/google-keyword-ranking-check/
Scraping from Google through python does have limits. Google has implemented several measures to limit scraping activities. These include rate limiting, CAPTCHAs, and IP blocking. Additionally, Google’s Terms of Service prohibits using automated methods to access their data, so scraping from Google may also be considered a violation of their terms.
You can use scraping API or proxies to bypass the limitations to enjoy the keyword ranking checker script for as many as keywords possible. I’m able to check 30-40 keywords in one go before Google blocks the IP to perform the searches on Google.
Output for checking rankings for mygreatlearning.com
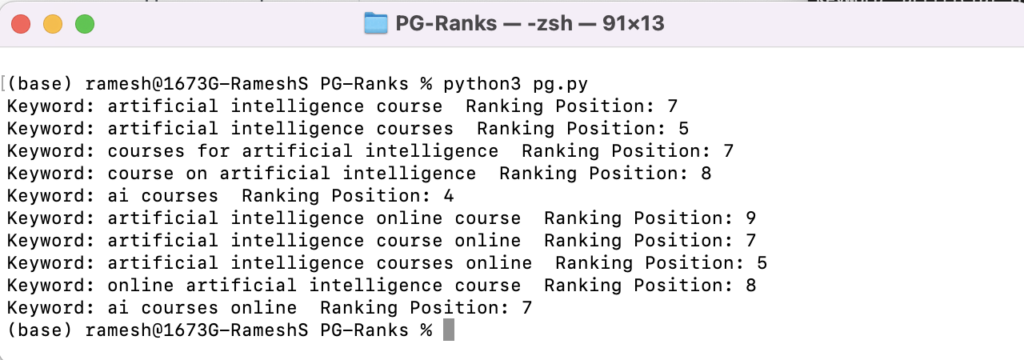
CSV Export of Ranking Data
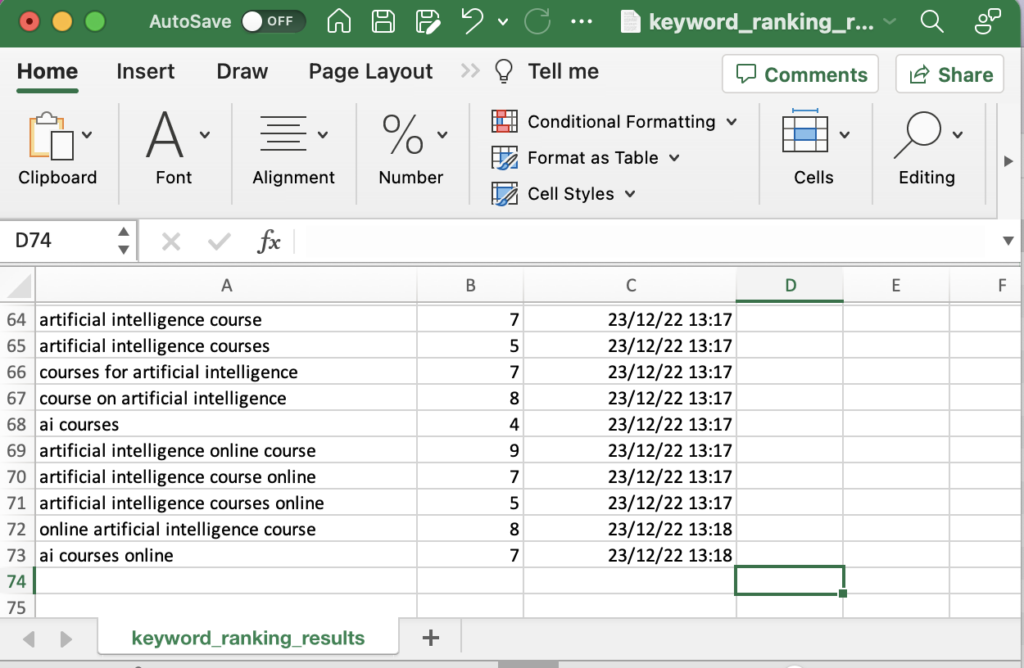
Disclamier: This article is for training purposes only and is not intended to be used as a tool for scraping data from Google. Scraping results from Google is a violation of the Google Terms of Service and can lead to legal problems. We do not condone scraping data from Google and this article is purely for training purposes to help SEO to automate processes.